say if I have an object at a certain position and i want to go to a certain position in another object by going around/rotating a pivot or another object.
right now, what i did is I linear interpolate the position every frame but the problem is it zooms inside the object which is not the effect I am looking,
example if the current camera position is at (0,0, -1) looking at the object at (0,0,0), then i want to go to (0,0,1) with linear interpolation, it will go thru inside the object. what i want is to rotate around that object.
i mean situation like the above is simple to figure out
i can just rotate the point around the pivot object center position (blue) and uses (0, 1, 0) as my axis as it only rotates around the Y axis.
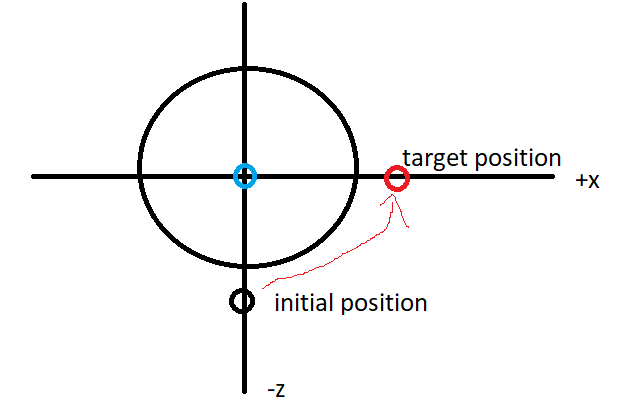
my problem is say the original position is somewhere with x, y and z values, that means i need to rotate it in all axis. such as this drawing
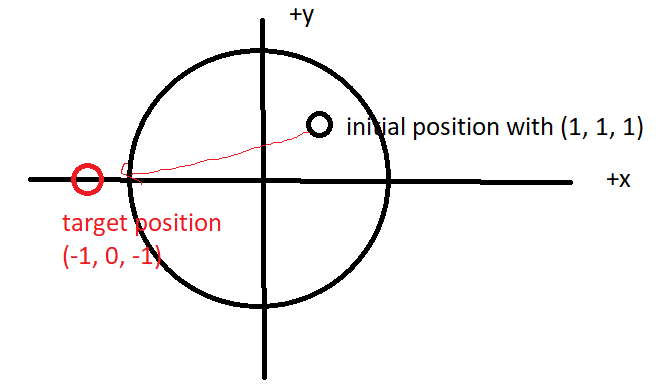
Now i need to figure out the axis to rotate to, and i am confuse on how to compute the axis to rotate to.
one idea i have is to get the difference between each axis, and use it as an angle and rotate them individualy using each axis.
angleX = targetX - origX
angleY = targetY - origY
angleZ = targetZ - origZ
new X position = rotate in axis (1,0,0) with angleX
new Y position = rotate in axis (0,1,0) with angleY
new Z position = rotate in axis (0,0,1) with angleZ
but the above results to new vector3 positions instead and kind of a bit hacky (i think).
Anyone got better or correct idea?