Hi
I have been using Bezier and convex hull libraries (C#) to try and make smooth border around rectangles and I'm trying use Quadratic bezier curves but the problem is I don't know how to calculate the middle control point.
Here's a screenshot of what I got so far (yellow line is convex hull, curves are the bezier):
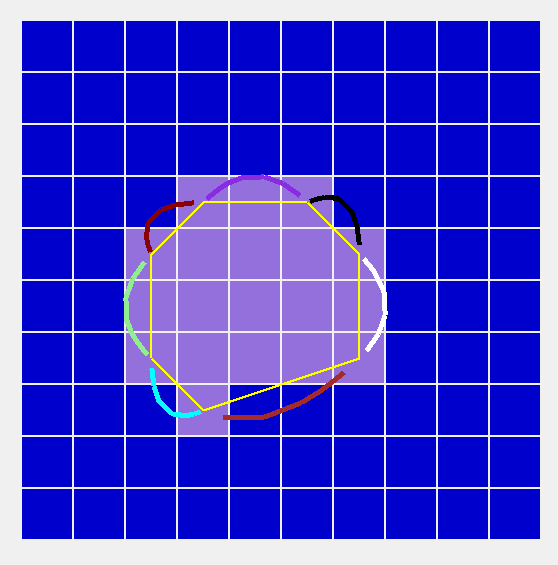
And here's the attemp to calculate that middle control point (ax,ay)
(sp is start point and ep end point)
double angle = Math.Atan2(((double)(ep.Y - sp.Y)),((double)(ep.X - sp.X))); // Count the direction from start point to end point
angle += Math.PI - (Math.PI * 0.25);
double length = 75.0;
int ax = (int)((Math.Sin(angle) * length));
int ay = -(int)((Math.Cos(angle) * length));
Bezier bez = new Bezier(new Vector2(sp.X, sp.Y), new Vector2(((sp.X + ax) ) , ((sp.Y + ay) ) ), new Vector2(ep.X, ep.Y));
Any tips are welcome but hopefully not heavy math formulas because I don't often understand those
thx!